Building a PDF-to-Podcast Pipeline with Open-Source AI: From Text Extraction to Voice Synthesis
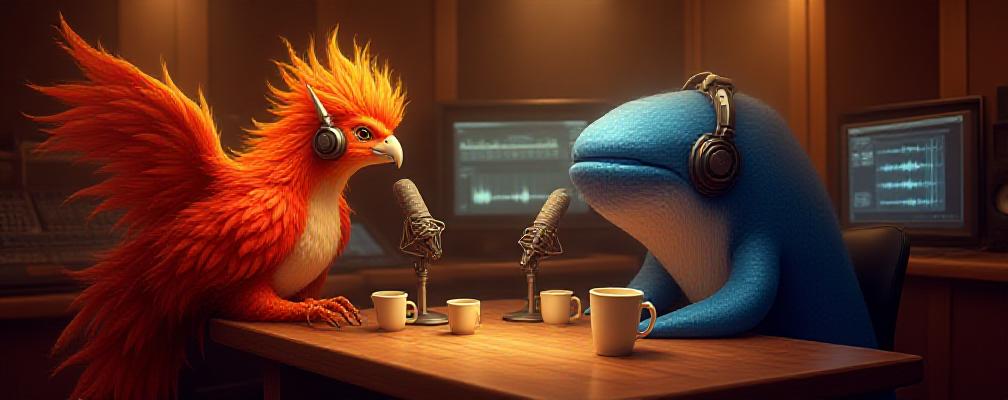
Introduction
Imagine this: you’re jogging through the park, earbuds in, grinning as two lively voices chat about the latest AI research paper, just like it’s a podcast made just for you. Or picture a busy content creator with a pile of blog posts, dreaming of turning them into audio gold without spending hours recording. That’s where this AI-powered pipeline comes in. It takes static PDFs and transforms them into engaging, conversational podcasts using open-source tools. In this post, I’ll walk you through the whole process: extracting text, crafting fun scripts, and synthesizing natural audio.
Why Turn PDFs into Podcasts?
PDFs are treasure troves of info, but let’s be real, they’re not exactly commute-friendly. Podcasts, though? They’re perfect for multitasking: driving, working out, or chilling out. The problem is, recording a podcast the old-school way: scripting, speaking, editing, is a time sink. This pipeline changes that. It automates the process, so you can focus on the content. Here’s who could use it:
Researchers: Turn dense papers into listens for your morning run.
Professionals: Make industry reports your gym-session soundtrack.
Bloggers: Repurpose old posts into fresh podcast episodes.
Technologies Used
The pipeline leverages several powerful open-source technologies:
PyMuPDF: For extracting text content from PDFs while preserving structure
Featherless.ai API: Access to all open-weight models on Hugging Face for text cleaning and creative podcast script generation by using roleplay finetunes.
Kokoro TTS: Converts text into natural-sounding audio.
Python Libraries: Tools like Pandas, NumPy, and PyDub handle data and audio processing.
The Complete Pipeline Overview
This pipeline architecture consists of four main stages:
Text Extraction and Cleaning: Converting PDF to structured, readable text
Podcast Script Generation: Transforming factual content into natural dialogue
TTS Optimization: Formatting the script for speech synthesis compatibility
Audio Generation: Creating and combining audio segments into a cohesive podcast
Let’s dive into each stage in detail.
The pipeline consists of four interconnected Jupyter notebooks, each handling a specific stage of the transformation process:
PDF Document → Text Extraction → Script Generation → TTS Optimization → Audio Generation
Stage 1: Text Extraction and Cleaning
Extracting text from PDFs with PyMuPDF
The first challenge is to extract text from PDF documents while preserving its meaning and structure. PDFs are notoriously difficult to parse correctly, as they can contain multiple columns, images, headers, footers, and complex layouts. I chose PyMuPDF (via the pymupdf4llm wrapper) for its ability to handle these complexities. Here’s the core extraction function:
def extract_text_from_pdf(file_path: str, max_chars: int = 60000) -> Optional[str]:
if not validate_pdf(file_path):
return None
try:
# Convert PDF to markdown text
markdown_text = pymupdf4llm.to_markdown(file_path)
# Truncate if exceeds max_chars
if len(markdown_text) > max_chars:
print(f"Truncating text to {max_chars} characters")
markdown_text = markdown_text[:max_chars]
print(f"\nExtraction complete! Total characters: {len(markdown_text)}")
return markdown_text
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
return None
What’s happening here? It checks the PDF’s legit, pulls text as Markdown to preserve structure (like headings), and trims it if it’s massive. For non-coders: this is like a super-smart photocopier that grabs only the words you care about. Watch out, though scanned PDFs or locked files might need some extra work.
Cleaning and Structuring Content
Raw PDF text is often cluttered with page numbers, headers, footers, and other elements that don’t belong in a podcast script. Plus, academic and technical documents frequently contain notation that doesn’t translate well to speech. I used the Featherless.ai API to process and clean this text. This approach leverages large language models to understand the content and reformat it appropriately:
def process_chunk(text_chunk, chunk_num):
"""Process a chunk of text using Featherless API"""
messages = [
{"role": "system", "content": SYS_PROMPT},
{"role": "user", "content": text_chunk},
]
try:
response = requests.post(
f"{BASE_URL}/chat/completions",
headers={
"Content-Type": "application/json",
"Authorization": f"Bearer {FEATHERLESS_API_KEY}"
},
json={
"model": DEFAULT_MODEL,
"messages": messages
}
)
response.raise_for_status()
processed_text = response.json()["choices"][0]["message"]["content"]
return processed_text
except Exception as e:
print(f"Error processing chunk {chunk_num}: {str(e)}")
return text_chunk # Return original text in case of error
The system prompt tells the model to keep the good stuff and ditch the rest:
```You are a world class text pre-processor, here is the raw data from a PDF,
please parse and return it in a way that is crispy and usable to send to a
podcast writer.
The raw data is messed up with new lines, LaTeX math and you will see fluff
that we can remove completely. Basically take away any details that you think
might be useless in a podcast author's transcript.
Remember, the podcast could be on any topic whatsoever so the issues listed
above are not exhaustive.
Please be smart with what you remove and be creative ok?
Remember DO NOT START SUMMARIZING THIS, YOU ARE ONLY CLEANING UP THE TEXT
AND RE-WRITING WHEN NEEDED.```
Example:
Before:
#Intro\nPage 1\nData is key\\LaTeX{math}
here.After: Data is key
Handling Technical Challenges
Big PDFs bring big challenges:
Memory Limits: Huge files can crash things, so I split text into 1,000-character chunks , like this:
Text → [Chunk 1 | Chunk 2 | Chunk 3] → Processed
.
Each chunk gets cleaned, then reassembled.Weird Layouts: PyMuPDF and the LLM team up to straighten out columns and tables so the flow makes sense.
The output of this stage is clean, well-structured text that captures the essential information from the PDF in a format suitable for conversion to podcast dialogue.
Stage 2: Podcast Script Generation
Next, we transform the text into a dialogue between two speakers using the Featherless.ai API and a large language model (LLM) of choice. It creates a natural back-and-forth:
Speaker 1: The explainer, dropping clear insights.
Speaker 2: The curious one, tossing in questions and quirks.
Here’s an example output:
SPEAKER 1: Data is critical for AI—it’s what powers the system, much like fuel for an engine.
SPEAKER 2: So, if the data isn’t great, does that affect how well the AI performs?
The LLM adds natural phrasing to make it feel like a real conversation, not just a read-aloud.
Stage 3: TTS Optimization
While the previous stage generated a conversational podcast script, this stage takes a different approach focused specifically on Text-to-Speech (TTS) compatibility. Instead of further processing the output from stage 2, we revisit the raw extracted text and apply specialized prompt engineering to generate a script format optimized for voice synthesis.
The Challenge of TTS-Ready Scripts
Text-to-speech engines often struggle with:
Natural-sounding dialogue that maintains distinct speaker voices
Appropriate pacing and pauses
Handling emotional expressions and reactions
Structured, predictable formats for programmatic processing
The goal of this stage is to transform our basic script into a structured format that both preserves its conversational nature and ensures reliable TTS processing as well as adds some flair to the conversation by using a specialized roleplaying language model accessed through Featherless.ai
SYSTEM_PROMPT = """
You are an international Oscar-winning screenwriter who has worked with
multiple award-winning podcasters.Your job is to rewrite the provided podcast
transcript for an AI Text-To-Speech pipeline.
The original transcript was written by a less experienced AI, so you need
to enhance it significantly.
Create an engaging dialogue between two speakers, each with distinct personalities:
- Speaker 1: A captivating teacher who leads the conversation,
explains concepts with vivid analogies and personal anecdotes,
and makes the topic accessible and memorable. They speak clearly and
confidently, without using filler words like "umm" or "hmm."
- Speaker 2: A curious and enthusiastic learner who keeps the conversation
on track by asking follow-up questions. They often get excited or confused,
expressing their reactions verbally with phrases like "That's fascinating!",
"Wait, I'm not sure I get that," or "Wow, that's like [analogy]."
[Additional instructions...]
Return the dialogue as a list of tuples, like this:
[
("Speaker 1", "Text here"),
("Speaker 2", "Text here"),
...
]
"""
This prompt engineers several crucial elements for TTS success:
Speaker-Specific Speech Patterns: By assigning distinct personalities, the model creates natural variations in speech patterns that TTS systems can interpret more distinctively.
Controlled Filler Usage: Speaker 1 avoids filler words while Speaker 2 can use them, creating natural rhythm without overwhelming the TTS engine.
Structured Data Format: The list of tuples creates a programming-friendly format that simplifies integration with TTS systems in the next stage.
By generating the script in this structured format, we eliminate many common TTS issues before they occur. The next stage can directly process this optimized script without additional parsing or formatting, streamlining the pipeline from text to spoken audio.
Stage 4: Audio Generation with Kokoro
The final stage transforms our TTS-optimized script into audio using Kokoro, an open-source text-to-speech library that provides high-quality voice synthesis.
Voice Selection and Configuration
Kokoro offers multiple voices with different characteristics. I selected distinct voices for each speaker to enhance the natural podcast feel:
# Initialize separate pipelines for each speaker with different voices
# Using American English as the base language
speaker1_pipeline = KPipeline(lang_code='a') # American English
speaker2_pipeline = KPipeline(lang_code='a') # American English
def generate_speech_kokoro(text, speaker="speaker1"):
# Select the appropriate pipeline and voice
if speaker == "speaker1":
# Use a female voice for Speaker 1
pipeline = speaker1_pipeline
voice = 'af_heart' # Female voice
speed = 1.0
else:
# Use a male voice for Speaker 2
pipeline = speaker2_pipeline
voice = 'am_fenrir' # Male voice
speed = 1.1 # Slightly faster
For our podcast, I chose:
Speaker 1:
af_heart
- Female American English voice with excellent qualitySpeaker 2:
am_fenrir
- Male American English voice with good quality
These different voices create a clear distinction between speakers, making the podcast easier to follow.
Combining Segments with Proper Timing
To create a cohesive podcast, we need to combine individual audio segments with appropriate spacing:
final_podcast = AudioSegment.empty()
for i, (speaker, text) in enumerate(tqdm(podcast_segments, desc="Generating podcast")):
speaker_id = "speaker1" if speaker == "Speaker 1" else "speaker2"
# Generate audio for this segment
audio_segment = generate_speech_kokoro(text, speaker_id)
if audio_segment:
# Add slight pause between segments
if i > 0:
final_podcast += AudioSegment.silent(duration=500) # 500ms pause
# Add to podcast
final_podcast += audio_segment
This code adds a half-second pause between speaker transitions, creating a natural rhythm in the conversation.
Challenges and Solutions
Building this pipeline wasn’t without hurdles. Here are some key challenges and how I tackled them:
Handling Complex PDF Layouts: PDFs with multi-column formats, images, or tables can be tricky. PyMuPDF’s Markdown conversion preserved some structure, but additional cleaning via the Featherless.ai API removed artifacts like page numbers and headers intelligently.
Generating Natural Dialogue: Turning static text into a dynamic conversation required careful prompt engineering. I guided the LLM to include interruptions, filler words, and personality-driven responses, making the script feel authentic.
Optimizing for TTS: Ensuring the script was TTS-friendly meant structuring it for easy synthesis. Using a tuple-based format and controlling filler usage prevented common TTS pitfalls, like unnatural pacing or mispronounced expressions.
Future Improvements
The pipeline works well, but there’s room to grow:
Multi-Language Support: Adding support for PDFs and podcasts in multiple languages would broaden its reach.
Advanced TTS Features: Integrating emotional tone adjustments or background music could make the podcasts more immersive.
Fine-Tuned Models: Using LLMs fine-tuned for podcast script generation could enhance dialogue quality further.
Try languages first, it’s a fun, doable leap and there are ton of finetuned models on Featherless.ai to assist you with that.
Conclusion
This PDF-to-podcast pipeline demonstrates the remarkable potential of open-source AI when creatively combined. By bridging PyMuPDF’s extraction capabilities with Featherless.ai’s language models and Kokoro’s voice synthesis, we’ve created a system that transforms static documents into engaging audio experience.
The true power lies in the modular design. Each component can be independently improved or replaced as new models emerge. Want to try a different LLM? Swap the API endpoint. Prefer different voices? Modify the TTS configuration. This flexibility makes it perfect for experimentation and customization.
We encourage readers to fork the project and make it their own. You can listen to a sample podcast generated with this pipeline or grab the full code on GitHub and start building your own. Try adding your own prompts, experiment with different voice combinations, or extend it to handle research papers and technical manuals. The future of content adaptation is open, accessible, and limited only by your imagination, happy podcasting!